Welcome to Flask-QRcode¶
Flask-QRcode is a concise Flask extension to easily render QR codes on Jinja2 templates using python-qrcode.
User’s guide¶
It is very straightforward to use Flask-QRcode on your Flask projects, and you can find a sample application with running examples on the repository on GitHub.
Installation¶
You can install it from PyPi:
$ pip install flask-qrcode
Or download the latest release from the GitHub repository.
Note tha all the latest releases should be signed with the PGP key 0x0dc77fc61ed3ecc4 (also found on keybase).
Basic usage¶
Extend the app:
from flask_qrcode import QRcode
# [...]
QRcode(app)
# [...]
Then use it within your templates:
{# Basic usage: #}
<img src="{{ qrcode(STRING_TO_ENCODE) }}">
More examples¶
Simple example:
<img src="{{ qrcode("Do you speak QR?") }}">
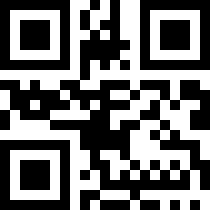
Changing box size:
<img src="{{ qrcode("I'm bigger than you!", box_size=20) }}">
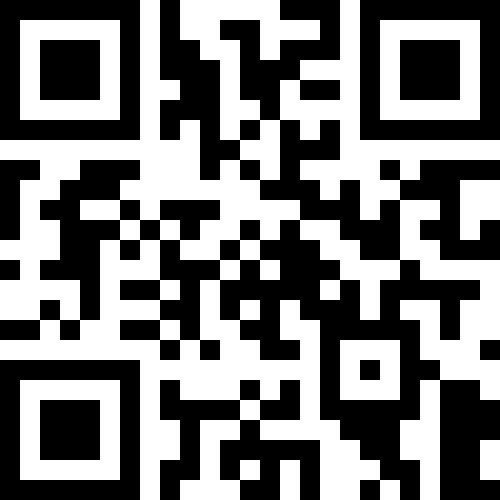
Changing border size:
<img src="{{ qrcode("Whatever... can't touch me", border=10) }}">
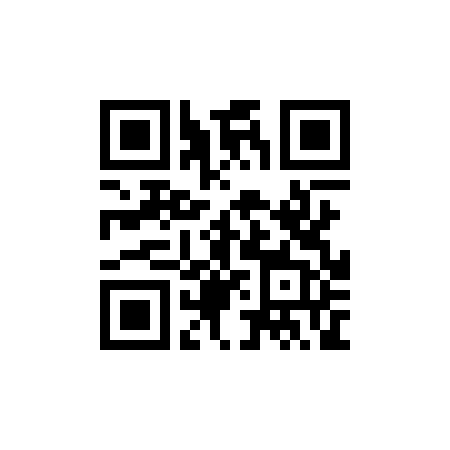
Changing QRcode error correction according to python-qrcode documentations:
<img src="{{ qrcode("You can read me even if I'm 30% hurt.", error_correction='H') }}">
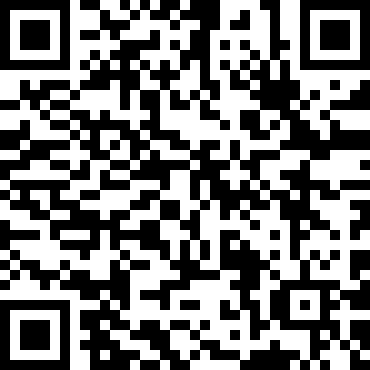
A “combo” example changing more than one parameter:
<img src="{{ qrcode("FATALITY", box_size=12, border=5) }}">
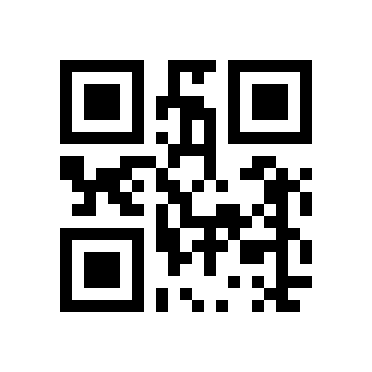
Changing the color of the QR code:
<img src="{{ qrcode("You can see green front color and #CCC back color.", error_correction='H', back_color='ccc', fill_color='green') }}">
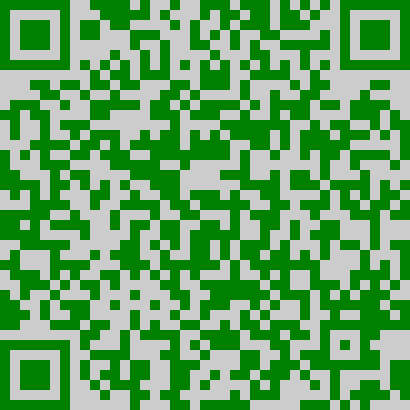
Rendering an icon inside the QR code (external link):
<img src="{{ qrcode("You can see a icon in QR code.", error_correction='H', icon_img='https://www.agner.io/icon.jpg') }}">
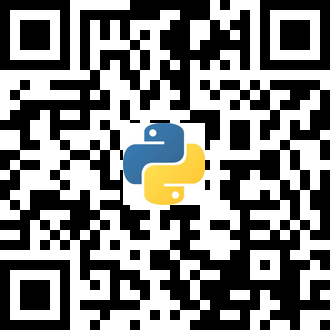
Rendering an icon inside the QR code (local file):
<img src="{{ qrcode("Load icon image from static folder.", error_correction='H', icon_img='icon.jpg') }}">
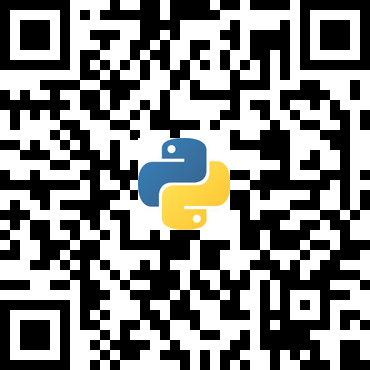
Contributing¶
Thank you for considering contributing to this package.
As this is a simple package, the process is pretty straightforward…
- Fork this repository
- Checkout from master with to a feature branch with a name related to what is being contributed (e.g. “colored-qrcodes”)
*It’s highly recommended that your contribution either creates a new feature, fixes something OR refactors the code and does not mix these (e.g. one PR fixing some existent feature and refactoring non-related code).
3. Install dependencies and flask_qrcode on editable mode
$ pip install -e . # for installing flask_qrcode on editable mode
- Do your magic
- Provide new tests for your work and check that both this and the old ones are passing.
- Pull Request!
Testing¶
1. Install dependencies and flask_qrcode on editable mode
$ pip install -e . # for installing flask_qrcode on editable mode
2. Run pytest
$ python setup.py test # in package's root dir
Source code documentation¶
// Automatically generated by Sphinx’s autodocs based on the source code’s docstrings.